Real-time Analytics
CrateDB provides a Unified Data Layer to ingest, index, and analyze vast amounts of data in real time, enabling you to make data-driven decisions and respond quickly to dynamic trends.
Want to discuss your real-time analytics projects?
Why CrateDB for real-time analytics?
Real-time query performance
Experience fast in-memory SQL query performance with CrateDB's parallel query processing and distributed columnar field caches. This feature enhances real-time analytics by optimizing query execution speed, ensuring swift data retrieval for immediate analysis and decision-making.
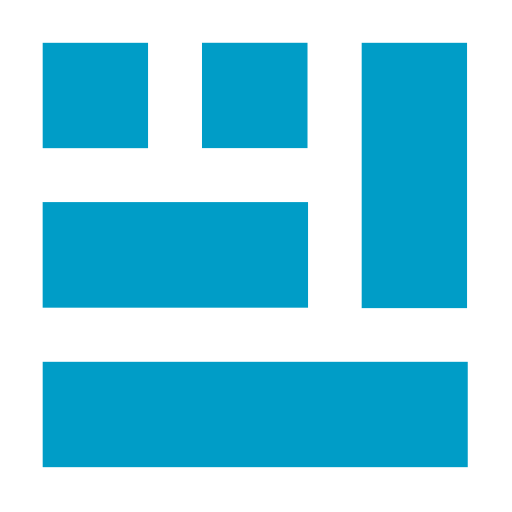
Horizontal scalability
Seamlessly scale CrateDB to manage the continuous influx of massive data from diverse sources. CrateDB's ability to scale horizontally across multiple nodes facilitates uninterrupted operations, allowing you to expand resources effortlessly to accommodate growing data needs, ensuring consistent performance for real-time analytics.
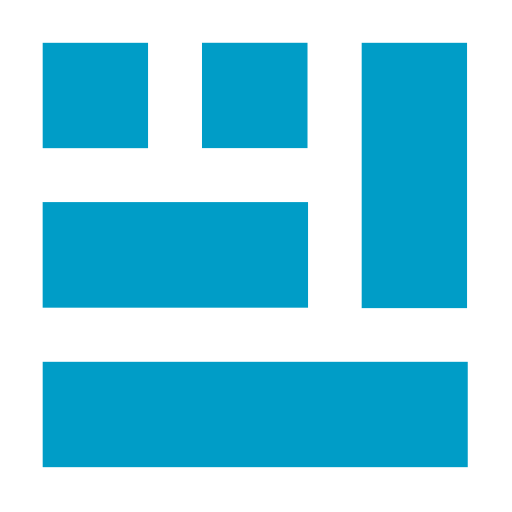
Distributed shared-nothing architecture
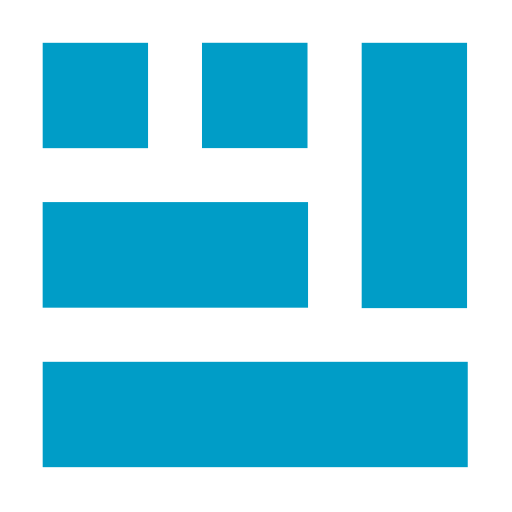
Real-time indexing
Ensure immediate data availability for queries, enabling swift access crucial for real-time monitoring, accelerated decision-making, and enhancing operational efficiency.
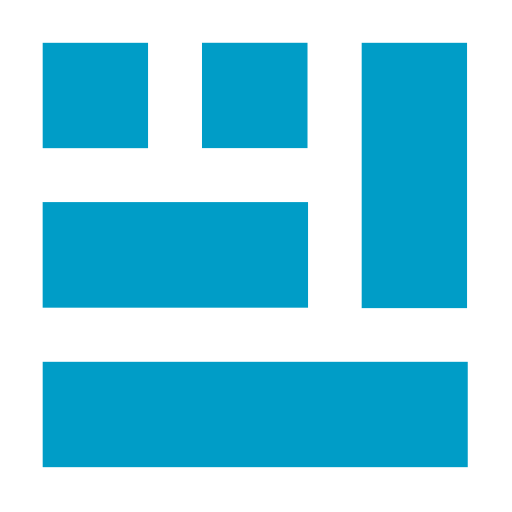
Fast data ingestion and processing
Ingest, store, and process millions of data points per second thanks to distributed processing, data partitioning, multithreaded design, and shared-nothing distributed architecture with masterless clustering.
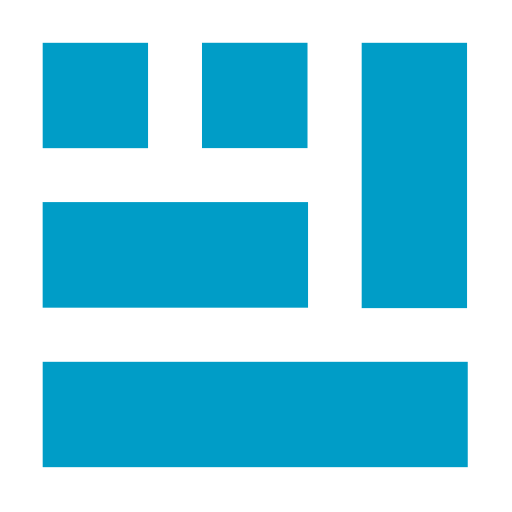
Built-in high availability
Ensure ultimate reliability and non-stop performance with automatic replication and self-healing. Instant availability of ingested data ensures immediate query access, delivering responses in milliseconds for intricate ad-hoc queries across vast datasets, ensuring real-time insights.
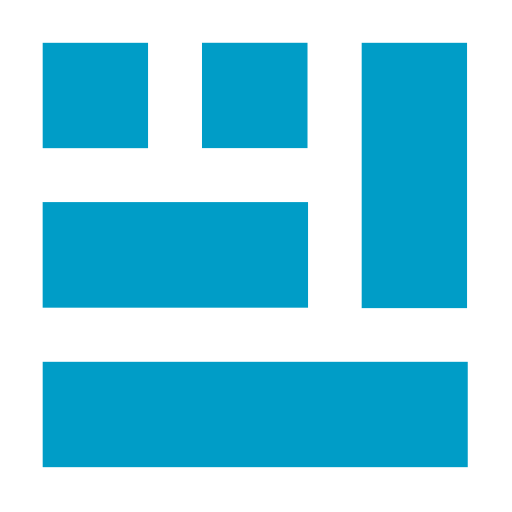
Columnar storage for data aggregations
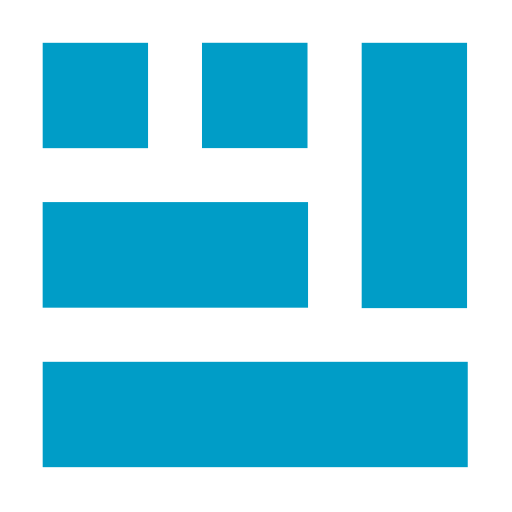
FAQ
A real-time analytics database is a database system designed to process and analyze data as it is generated, providing insights and results in real-time. Some examples of databases for real-time analytics include CrateDB, SingleStore, StarTree, and InfluxDB. CrateDB excels in ingesting, indexing, storing, and querying large amounts of data within milliseconds, enabling organizations to make data-driven decisions and respond to dynamic trends quickly.
Real-time data refers to information that is gathered and processed immediately upon being generated. Examples include data from social media feeds, live customer interactions, and IoT devices. CrateDB's flexible data modeling allows for the collection and storage of a wide range of data types from diverse sources, such as enterprise applications, analytics platforms, and sensor networks.
Real-time data collection can be achieved through various methods such as streaming data ingestion, API integrations, or real-time data capture tools. CrateDB supports fast data ingestion and processing, handling millions of data points per second.
Real-time data is typically stored in databases or high-speed data stores designed to handle rapid data processing. CrateDB utilizes columnar storage to compute data aggregations on demand without downsampling or pre-aggregation, facilitating dynamic and immediate analysis of large datasets.
An example of real-time analysis could be a live dashboard displaying website visitor behavior or a system monitoring tool tracking server performance in real-time. Learn how O-CELL's real-time monitoring solution helps reduce the environmental impact with CrateDB >